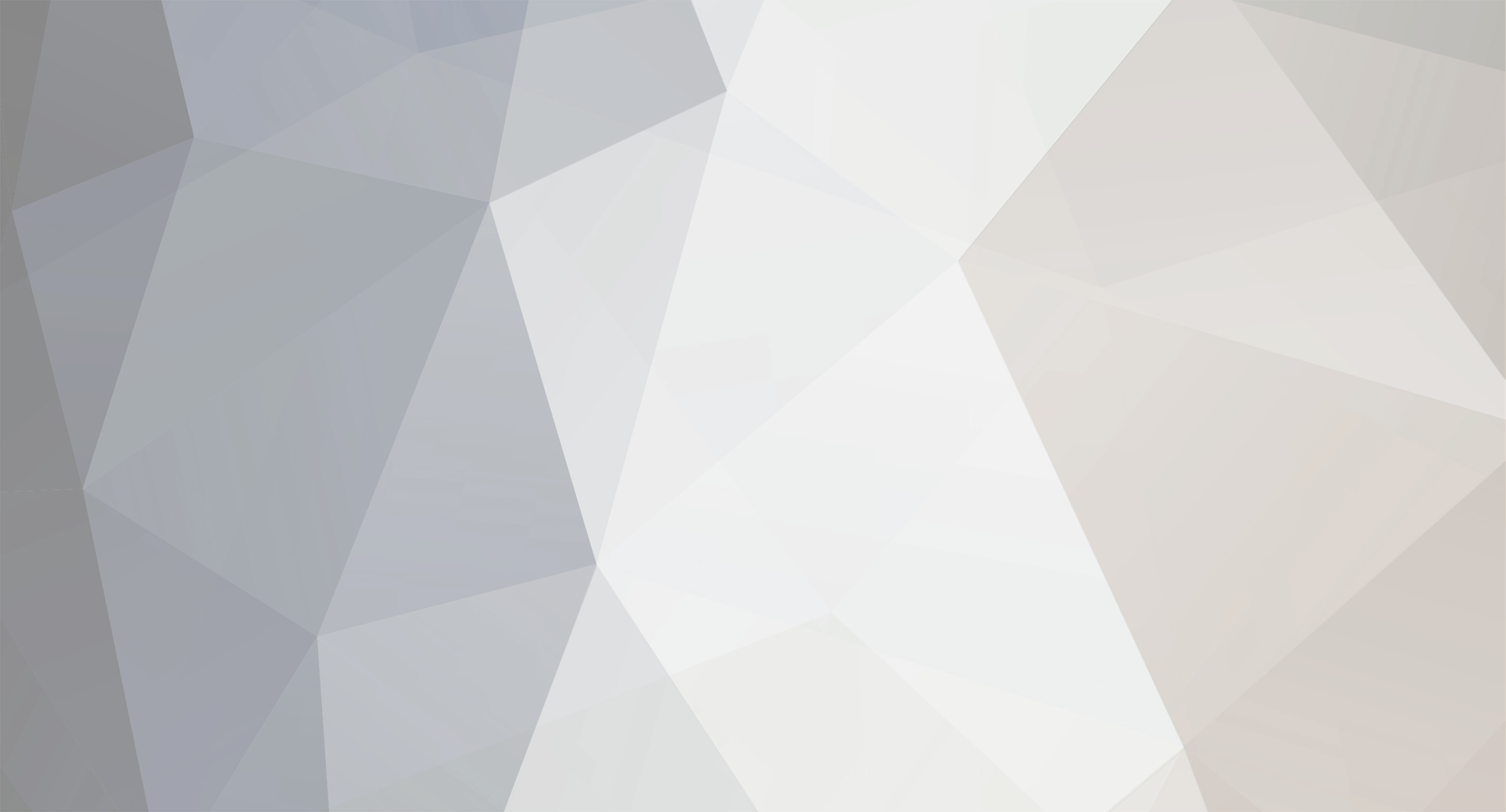
loopyc
-
Posts
54 -
Joined
-
Last visited
Reputation Activity
-
loopyc reacted to AM in gen-brownian-bridge
something new...
greetings
andré
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; ;;; BROWNIAN BRIDGE -> could be use as a rnd-process from A to B (integers or pitches) ;;; if you have a look to example with ":all-gen t", you will see the process with all generations, how it works ;;; or take a look to: ;;; https://de.wikipedia.org/wiki/Wiener-Prozess#/media/File:BrownscheBewegung.png ;;; https://de.wikipedia.org/wiki/Brownsche_Brücke ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; ;;; SUB (defun pick (a b &key (span 5)) (let ((rnd1 (car (rnd-number 1 (+ a span) (- a span)))) (rnd2 (car (rnd-number 1 (+ b span) (- b span)))) (n)) (progn (setf n (car (rnd-number 1 rnd1 rnd2))) (if (or (= n a) (= n b)) (+ (rnd-pick '(1 -1)) n) n)))) ;;; MAIN ;;; MAIN (defun gen-brownian-bridge (n startend &key (all-gen nil) (output 'integer) (span 5)) (let ((seq)) (progn (setf seq (append (list startend) (loop repeat n with liste = startend do (setf liste (filter-repeat 1 (loop repeat (1- (length liste)) for cnt = 0 then (incf cnt) append (append (list (nth cnt liste) (pick (nth cnt liste) (nth (1+ cnt) liste) :span span) (nth (1+ cnt) liste)))))) collect liste))) (setf seq (if (equal all-gen t) seq (car (last seq)))) (if (equal output 'pitch) (integer-to-pitch seq) seq)))) ;;; EXAMPLES ;; SPAN influence -> span 2 (list-plot (gen-brownian-bridge 5 '(50 23) :span 2 :all-gen t) :zero-based t :point-radius 3 :join-points t) ;; SPAN influence -> span 10 (list-plot (gen-brownian-bridge 5 '(50 23) :span 20 :all-gen t) :zero-based t :point-radius 3 :join-points t) ;;; SPAN default (5) (list-plot (gen-brownian-bridge 5 '(50 23) :all-gen t) :zero-based t :point-radius 3 :join-points t) (list-plot (gen-brownian-bridge 5 '(50 23)) :zero-based t :point-radius 3 :join-points t) (gen-brownian-bridge 5 '(50 23) :all-gen t :output 'pitch) (gen-brownian-bridge 5 '(50 23) :output 'pitch)
some sound-examples
;;; EXAMPLE with ALL GENS / seperated by rests (def-score brownian-bridge (:title "score title" :key-signature 'atonal :time-signature '(4 4) :tempo 72) (instrument :omn (make-omn :pitch (setf n (gen-brownian-bridge 5 '(30 10) :all-gen t :output 'pitch)) :length (loop for i in n append (list '-1/4 (loop repeat (length i) append '(t)))) :span :pitch) :channel 1 :sound 'gm :program 'acoustic-grand-piano)) ;;; EXAMPLE with LAST GEN -> rnd-evaluations => rnd-ways from a to b (def-score brownian-bridge (:title "score title" :key-signature 'atonal :time-signature '(4 4) :tempo 72) (instrument :omn (make-omn :pitch (gen-brownian-bridge (car (rnd-number 1 2 7)) '(30 10) :output 'pitch) :length '(t) :span :pitch) :channel 1 :sound 'gm :program 'acoustic-grand-piano))
two examples with different SPAN on MESSIEAN's mode5 mapped with <tonality-map>
;;; on MESSIAENS- mode5 - > 8 cycles + SPAN 10 => bigger intervals/steps (def-score brownian-bridge (:title "score title" :key-signature 'atonal :time-signature '(4 4) :tempo 90) (instrument :omn (make-omn :pitch (setf n (tonality-map '(messiaen-mode5 :map step :root 'fs3) (integer-to-pitch (gen-brownian-bridge 8 '(10 27) :span 10 :all-gen t)))) :length (loop for i in n append (list '-1/4 (loop repeat (length i) append '(t)))) :span :pitch) :channel 1 :sound 'gm :program 'acoustic-grand-piano)) ;;; on MESSIAENS- mode5 - > 8 cycles + SPAN 3 => smaller intervals/steps (def-score brownian-bridge (:title "score title" :key-signature 'atonal :time-signature '(4 4) :tempo 90) (instrument :omn (make-omn :pitch (setf n (tonality-map '(messiaen-mode5 :map step :root 'fs3) (integer-to-pitch (gen-brownian-bridge 8 '(10 27) :span 3 :all-gen t)))) :length (loop for i in n append (list '-1/4 (loop repeat (length i) append '(t)))) :span :pitch) :channel 1 :sound 'gm :program 'acoustic-grand-piano))
-
loopyc reacted to opmo in sorting algorithms
A random sort '? - RND-PICK from ascending and descending result of a sequence.
Ascending:
(list-plot (flatten (sorting (rnd-number 20 1 10 :seed 2346) :type 'selection :sort '<)) :zero-based t :point-radius 1 :join-points t)
Descending:
(list-plot (flatten (sorting (rnd-number 20 1 10 :seed 2346) :type 'selection :sort '>)) :zero-based t :point-radius 1 :join-points t)
At random:
(list-plot (flatten (sorting (rnd-number 20 1 10 :seed 2346) :type 'selection :sort '?)) :zero-based t :point-radius 1 :join-points t)
In 5 steps:
(list-plot (flatten (sorting (rnd-number 20 1 10 :seed 2346) :type 'selection :sort '? :step 5)) :zero-based t :point-radius 1 :join-points t)
The default sort is ascending.
-
loopyc reacted to torstenanders in sorting algorithms
Simply slightly edit the algorithms below by inserting some additional output. Below I simply took one of the algorithms you linked and added a single print statement. If instead you want to collect all the results, then just accumulate them in some variable with a scope surrounding your algorithm function.
(defun bubble-sort/naive (sequence) (let ((end (length sequence))) (labels ((compare-and-swap (index modified) ;; print intermediate results (print sequence) (if (= index (1- end)) (if modified (compare-and-swap 0 nil) (values)) (let ((index+1 (1+ index))) (if (> (elt sequence index) (elt sequence index+1)) (let ((x (elt sequence index))) (setf (elt sequence index) (elt sequence index+1) (elt sequence index+1) x) (compare-and-swap index+1 t)) (compare-and-swap index+1 modified)))))) (unless (< end 2) (compare-and-swap 0 nil)) sequence))) (bubble-sort/naive '(3 1 9 5 3 6 4 2 3 7))
Best,
Torsten
-
loopyc reacted to AM in sorting algorithms
i solved the problems. here is a workspace/files to experiment with SORTING ALGORITHMS
thanx to torsten and philippe!
greetings
andré
functions.opmo
abstract examples.opmo
sound examples.opmo
sorting algorithms.opmows
-
loopyc reacted to opmo in length-to-decimal / length-to-sec
I used this function for conversion of 4 instrument each with in its own tempo to one 'global' tempo.
Examples:
(length-tempo-map 72 36 '1/4) => 1/2 (length-tempo-map 72 36 'w) => 2 (length-tempo-map 72 88 '(1/8 1/4 -1/4)) => (9/88 9/44 -9/44) (length-tempo-map 72 '(120 36) '((1/8 1/4 -1/4) (1/16 1/8 -1/4 1/1))) => ((3/40 3/20 -3/20) (1/8 1/4 -1/2 2)) (length-tempo-map 72 '(36 96 72) '((1/20 1/20 1/20 1/20 1/20) (1/16 1/16 1/16 1/16 1/16 1/16 1/16 1/16) (1/8 1/8 1/8 1/8) (1/4 1/4 1/4 1/4 1/4 1/4 1/4 1/4)) :meter '(1 1 1)) => ((1/10 1/10 1/10 1/10 1/10) (1/8 1/8 1/8 1/8 1/8 1/8 1/8 1/8) (1/4 1/4 3/32 3/32) (3/16 3/16 3/16 1/4 1/4 1/4 1/4 1/2)) (length-tempo-map 72 36 '((e c4 e4 g4 h_e c5) (q c4 = = - - =) (q cs5 = - - = =))) => ((q c4 e4 g4 w_q c5) (h c4 = = - - =) (h cs5 = - - = =)) (length-tempo-map 72 '(36 96 72) '((e c4 e4 g4 h_e c5) (q c4 = = - - =) (q cs5 = - - = =)) :meter '(1 1 1)) => ((q c4 e4 g4 w_q c5) (e. c4 = = - -q =) (q cs5 = -h - = =)) -
loopyc reacted to AM in length-to-decimal / length-to-sec
two functions i needed for working with POLYTEMPO-NETWORK
http://philippekocher.ch/#109
http://polytempo.zhdk.ch
greetings
andré
(defun length-to-decimal (alist &key (sum nil)) (let ((list (loop for i in (omn :length alist) collect (float (* i 4))))) (if (equal sum t) (sum list) list))) ;;; result: q = 1 / h. = 3 ...etc... (length-to-decimal '(h. h. 3q 5e 3h)) => (3.0 3.0 0.33333334 0.1 0.6666667) (length-to-decimal '(h. h. 3q 5e 3h) :sum t) => 7.1 (defun length-to-sec (alist tempo &key (sum nil)) (let ((list (loop for i in (omn :length alist) collect (* (/ 60 tempo) (float (* i 4)))))) (if (equal sum t) (sum list) list))) (length-to-sec '(h. h. 3q 5e 3h) 60) => (3.0 3.0 0.33333334 0.1 0.6666667) (length-to-sec '(h. h. 3q 5e 3h) 51) => (3.5294118 3.5294118 0.3921569 0.11764707 0.7843138) (length-to-sec '(h. h. 3q 5e 3h) 51 :sum t) => 8.3529415
-
loopyc reacted to opmo in Loading a pre-compiled midi-file?
This is quite simple to do, just use the pathname (string) to your midi file:
(live-coding-midi "~/Opusmodus/Media/MIDI/Handel/handel.mid")
-
-
-
loopyc reacted to opmo in Saving Plots and Piano Rolls to PDF Vectorial Graphics ETC
The multi screen support will be part of ver. 2.0
-
loopyc reacted to opmo in euclidean to interval
PATTERN-MAP
(pattern-map '((1 0) 2) (gen-binary-euclidean 1 14 8 8)) => (2 1 2 2 2 1 2 2)
-
loopyc reacted to Stephane Boussuge in November 13th Strings Trio
Score files updated with missing functions 1/F-values and euclidean-rhythms.
SB.
-
loopyc reacted to Stephane Boussuge in Modelling Tonality (1) Diatonic Transposition (some intuitions)
Here's my way for diatonic transposition.
It is very simple but do exactly what i want when composing
I use this system extensively in all my compositions now, not always in diatonic context but also with synthetic modes, row, algorithmic pitch material etc...
I love the concept of degree and transpositions inside a scale and use that technique very often.
(setf motiv '((q c4 e4 g4)(q c4 e4 g4)(q c4 e4 g4)(q c4 e4 g4)(q c4 e4 g4))) (setf degree '(1 4 2 5 1)) (setf harmonic-path (harmonic-progression degree '(c4 major) :step 1 :size 7 :base 1 )) (setf p1 (tonality-map (mclist harmonic-path) motiv))
S.
-
loopyc reacted to JulioHerrlein in Combinatorial Voice-Leading of Hexachords
Dear All,
HAPPY 2018 !!
With the new PCS organization in Opusmodus is possible to implement a concept of my book, called Combinatorial Voiceleading of Hexachords.
From a Hexachord Set, is possible to find 10 different ways to combine the notes in the for of voice-leading sets. Each hexachord is divided in (3 + 3) way.
This expression:
(setf hexavl (mclist (chordize-list (integer-to-pitch (remove-duplicates (sort-asc (gen-divide 3 (flatten (permute (pcs '6-32))))) :test #'equal)))))
Will result in this combination of the 6-32 hexachord, similar to the idea in the book.
In the book, the material is organized in 70 pages of melodic and harmonic exercises.
Here is a litte sample:
CH_HERRLEIN.pdf
The entire book:
https://www.melbay.com/Products/Default.aspx?bookid=30042BCDEB
Best !
Julio Herrlein
-
loopyc got a reaction from JulioHerrlein in Pcs: how to retrieve inverted forms from this function ?
I opted for a 'physical copy', so will have to wait until Dec. 21st...just in time for a Christmas gift to myself ;-)
-
loopyc got a reaction from JulioHerrlein in Pcs: how to retrieve inverted forms from this function ?
Going to, looks good...thanks for the notice ;-)
-
loopyc reacted to JulioHerrlein in Pcs: how to retrieve inverted forms from this function ?
WOW !!! GREAT !
Thanks !
It was really a lot of work done !
Lots of sets, but it's really useful, I think.
Best !
Julio
This will be in the next release, I think. Looking Forward !
added 2 minutes later If you like Set Theory and Guitar, you gonna like my book. Please, Check it out !
https://www.melbay.com/Products/Default.aspx?bookid=30042BCDEB
Best,
Julio
-
loopyc reacted to opmo in tutorial guide
Next - which will take some time - I will add all Opusmodus System Function with examples to our forum.
-
loopyc got a reaction from torstenanders in tutorial guide
SB:
https://opusmodus.com/forums/tutorials/tutorial-guide/
-
-
loopyc reacted to JulioHerrlein in Pcs: how to retrieve inverted forms from this function ?
It's a really interesting issue to study the different approaches of the post tonal Theory. The more hardcore atonal side does not consider prime and inverted form as different things, ontologically but in fact, our tonal oriented ear really can tell the difference between a major and a minor triad as a clear episthemological distinction.
Counting or not counting the inverted forms also have implications on the Problems of enumeration of the types of series, as Frippertinger & Lackner recent studies related to group theory and a more modern approach to Hauer Tropes demonstrates.
Best
Julio
-
loopyc reacted to JulioHerrlein in Pcs: how to retrieve inverted forms from this function ?
Very cool, Janusz!
Im doing my doctoral dissertation now and I'm doing some thing interesting related to rhythm that certainly could be transformed in a function. I'll tell you soon. This Will be useful there.
Best
Julio
-
loopyc reacted to opmo in Pcs: how to retrieve inverted forms from this function ?
I will add the inverted form into to the system with the next update.
-
loopyc reacted to opmo in Opusmodus 1.2.22733
Extended documentation and bug fix in do-timeline and do-timline2 functions if binary list.
-
loopyc reacted to torstenanders in Translating MIDI velocities into OMN velocities
Ah, great!
The documentation does not mention that the input can also be MIDI velocity integers, so I missed that.
Best,
Torsten
added 2 minutes later BTW: I needed that to translate values from OpenMusic data objects into Opusmodus. I am currently working on a library that will bring OpenMusic functions/methods to Opusmodus...
Best,
Torsten